Video
Delay
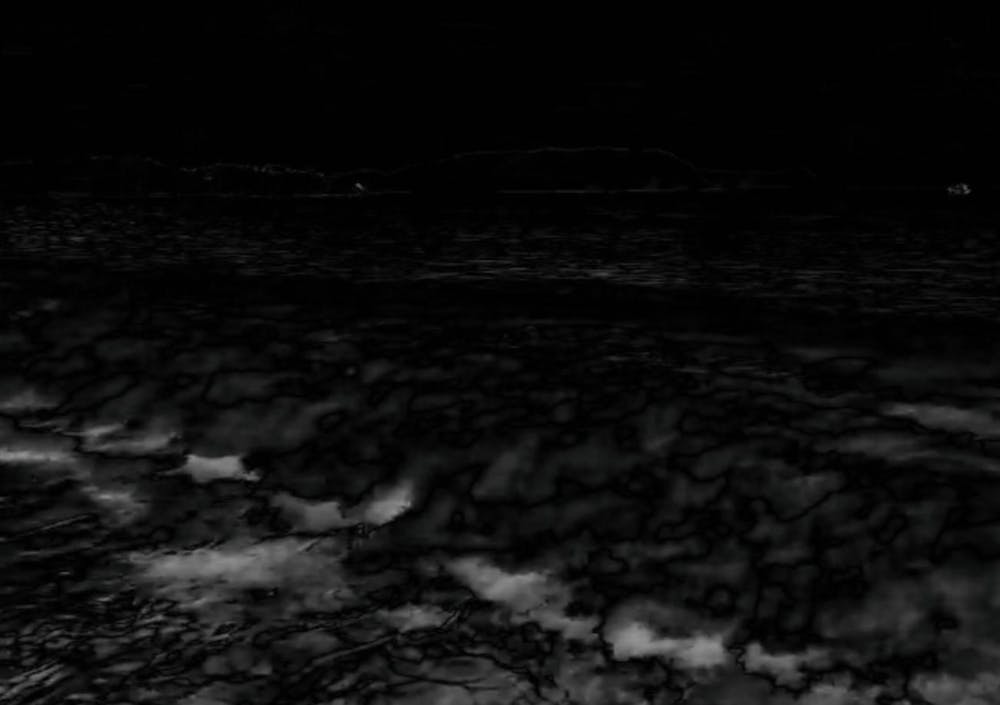
Inspired by this video by Posy. I thought it could be done automatically with OpenCV.
A lot of the commonets on the video say that it's similar to motion amplification and this is not that. This is much simplier, the motion amplification actually finds frequrencies of motion in the video and amplifies them, all this is doing is finding the difference between two frame.
As he explains in the video, what he is doing is performing a diff between the current frame and previous frame. This methodoly is very common in computer vision so I created an example app to demo it in Vue 3 and OpenCV.js.
The major functioning part of the code is this
// renderes the current frame of video to a canvas so it can be read ctx.drawImage(video, 0, 0, canvas.width, canvas.height); const mat = cv.imread(canvas); // save the video frame, so we can use it later const forColor = mat.clone(); // make the image grey scale and add it to the buffer cv.cvtColor(mat, mat, cv.COLOR_RGBA2GRAY); buffer.push(mat); // clean up the buffer so it doesn't get too long if (buffer.length > props.bufferSize) { buffer[0].delete(); buffer.shift(); } if (buffer.length > 1) { const diff = buffer[0].clone(); // find the difference between the last frame and first cv.absdiff(buffer[0], buffer[buffer.length - 1], diff); if (props.showMask) { // convert the image back to colour so we can render it cv.cvtColor(diff, diff, cv.COLOR_GRAY2RGBA); cv.add(forColor, diff, diff); } // render it cv.imshow(out, diff); // clean up diff.delete(); } forColor.delete();